why do i draw 3d boxes
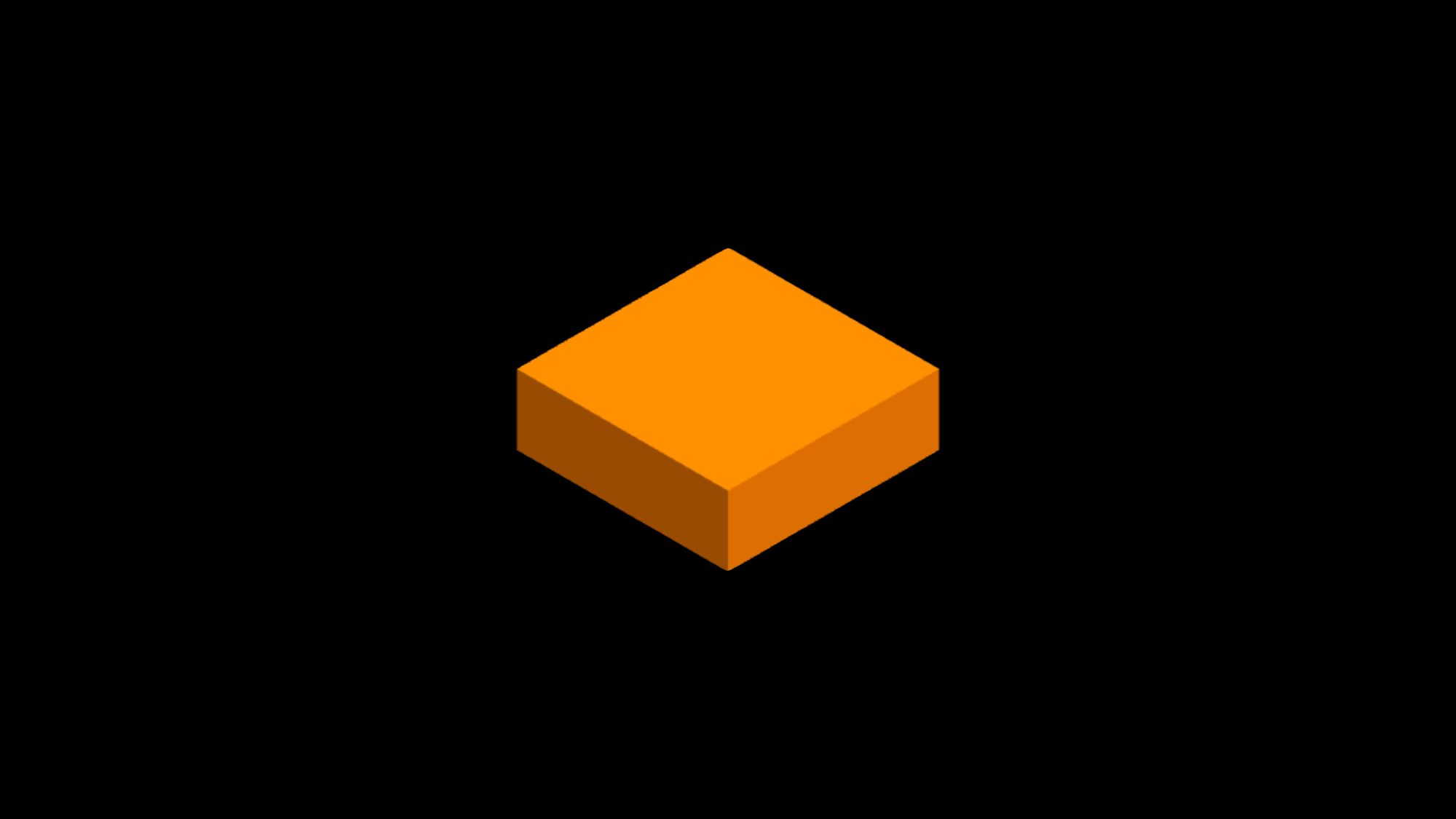
If you have ever wanted to build a game with JavaScript, you might take come across Three.js.
Three.js is a library that we can utilize to render 3D graphics in the browser. The whole matter is in JavaScript, and so with some logic yous can add blitheness, interaction, or even plow it into a game.
In this tutorial, we will go through a very simple example. We'll return a 3D box, and while doing and then we'll learn the fundamentals of Three.js.
3.js uses WebGL under the hood to render 3D graphics. We could use plain WebGL, merely it's very complex and rather depression level. On the other hand, 3.js is like playing with Legos.
In this commodity, we'll get through how to place a 3D object in a scene, prepare up the lighting and a camera, and render the scene on a canvas. So let'southward see how we can do all this.
Define the Scene Object
Start, we have to define a scene. This will be a container where we place our 3D objects and lights. The scene object likewise has some properties, similar the background color. Setting that is optional though. If nosotros don't ready it, the default will be blackness.
import * as THREE from "3"; const scene = new THREE.Scene(); scene.background = new THREE.Colour(0x000000); // Optional, black is default ...
Geometry + Fabric = Mesh
And then we add together our 3D box to the scene as a mesh. A mesh is a combination of a geometry and a textile.
... // Add a cube to the scene const geometry = new 3.BoxGeometry(3, 1, 3); // width, peak, depth const fabric = new Three.MeshLambertMaterial({ color: 0xfb8e00 }); const mesh = new Three.Mesh(geometry, textile); mesh.position.prepare(0, 0, 0); // Optional, 0,0,0 is the default scene.add(mesh); ...
What is a Geometry?
A geometry is a rendered shape that nosotros're building - like a box. A geometry can be build from vertices or we tin can use a predefined i.
The BoxGeometry is the near basic predefined option. We only have to set the width, summit, and depth of the box and that's it.
You might retrieve that we can't get far by defining boxes, but many games with minimalistic design utilize only a combination of boxes.
In that location are other predefined geometries likewise. We tin easily define a plane, a cylinder, a sphere, or even an icosahedron.
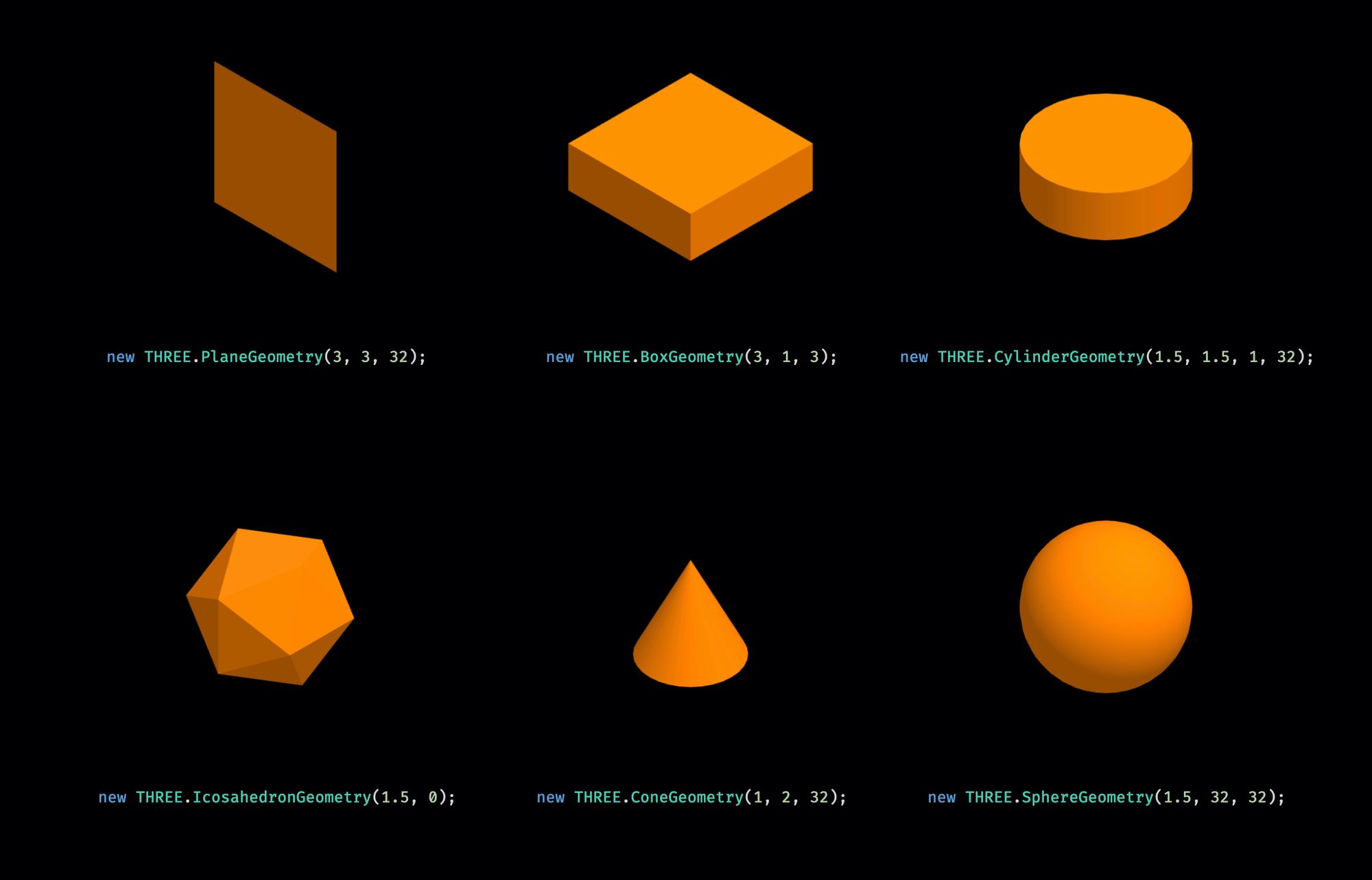
How to Work with Fabric
Then we define a textile. A material describes the appearance of an object. Here we can define things similar texture, color, or opacity.
In this case we are only going to set a color. At that place are yet different options for materials. The main departure between nigh of them is how they react to light.
The simplest one is the MeshBasicMaterial. This material doesn't care about calorie-free at all, and each side will have the aforementioned color. It might not be the best option, though, as yous can't see the edges of the box.
The simplest material that cares about light is the MeshLambertMaterial. This will summate the color of each vertex, which is practically each side. Simply it doesn't go beyond that.
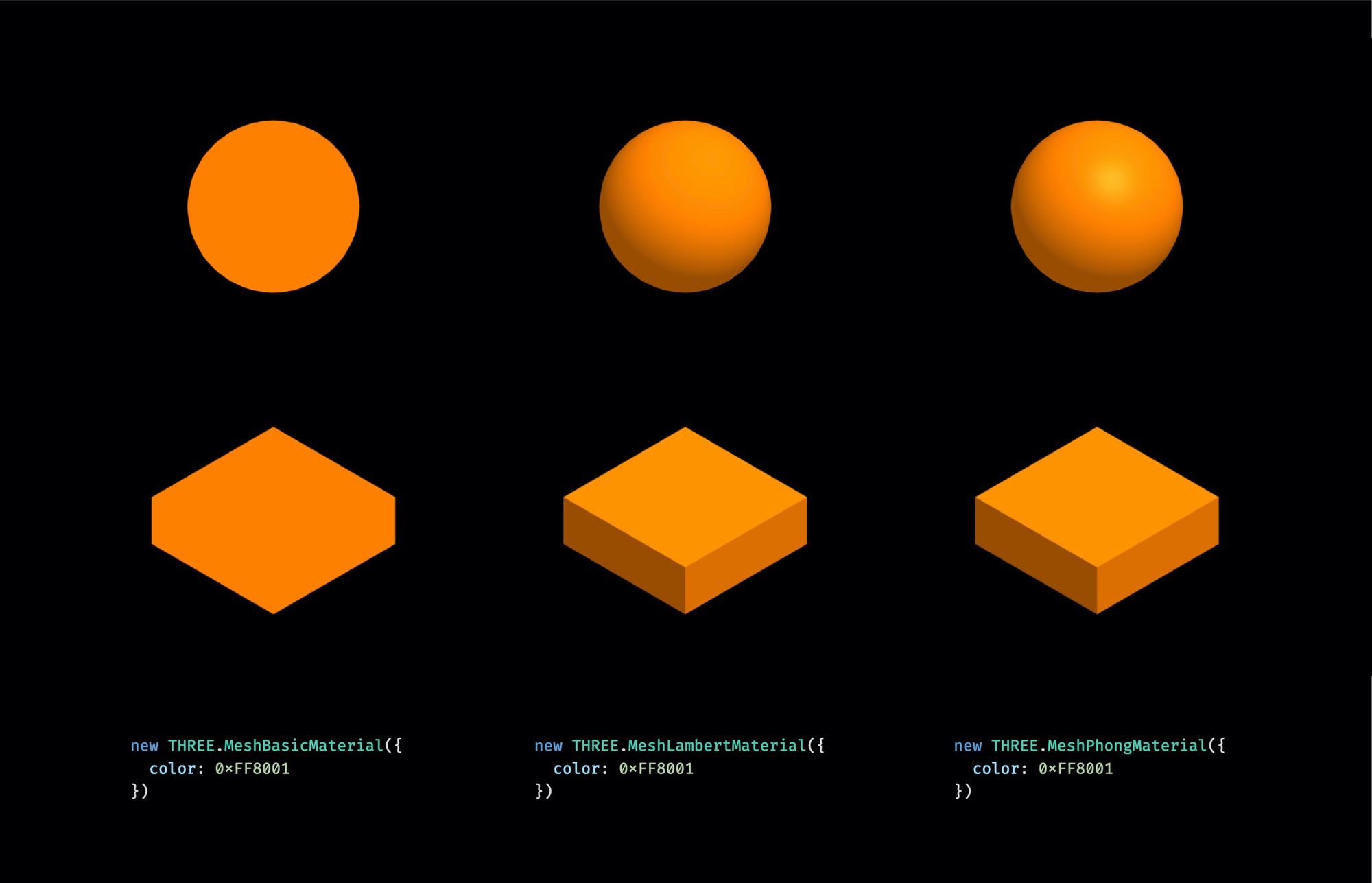
If you need more precision, there are more than advanced materials. The MeshPhongMaterial not only calculates the color by vertex but by each pixel. The colour tin can modify within a side. This tin can help with realism but also costs in performance.
Information technology also depends on the light settings and the geometry if it has any real effect. If we render boxes and use directional light, the result won't modify that much. But if nosotros render a sphere, the departure is more than obvious.
How to Position a Mesh
Once we have a mesh nosotros can besides position it within the scene and gear up a rotation by each axis. Later if we want to breathing objects in the 3D space we will more often than not adjust these values.
For positioning we employ the aforementioned units that we used for setting the size. It doesn't thing if yous are using small numbers or big numbers, you lot just demand to be consequent in your own world.
For the rotation we set the values in radians. Then if you take your values in degrees y'all have to divide them by 180° then multiply by PI.

How to Add Low-cal
Then let'southward add lights. A mesh with basic textile doesn't demand any calorie-free, as the mesh will have the set color regardless of the lite settings.
But the Lambert fabric and Phong material require light. If in that location isn't any light, the mesh volition remain in darkness.
... // Ready upwards lights const ambientLight = new THREE.AmbientLight(0xffffff, 0.half-dozen); scene.add together(ambientLight); ...
We'll add two lights - an ambience calorie-free and a directional lite.
First, we add the ambient calorie-free. The ambient light is shining from every direction, giving a base of operations color for our geometry.
To prepare an ambience light we set a color and an intensity. The color is usually white, but you tin set any color. The intensity is a number between 0 and 1. The two lights we ascertain piece of work in an accumulative mode then in this example we want the intensity to be effectually 0.5 for each.

The directional low-cal has a like setup, but it also has a position. The word position here is a bit misleading, because it doesn't mean that the light is coming from an exact position.
The directional light is shining from very far away with many parallel lite rays all having a stock-still angle. But instead of defining angles, we define the direction of a single calorie-free ray.
In this instance, it shines from the direction of the x,twenty,0 position towards the 0,0,0 coordinate. But of course, the directional calorie-free is not only i light ray, but an infinite amount of parallel rays.
Call up of information technology as the sunday. On a smaller calibration, calorie-free rays of the sun also come up down in parallel, and the sun's position isn't what matters but rather its direction.
And that's what the directional light is doing. It shines on everything with parallel light rays from very far away.
... const dirLight = new 3.DirectionalLight(0xffffff, 0.6); dirLight.position.set up(10, twenty, 0); // x, y, z scene.add together(dirLight); ...
Here we set the position of the light to be from in a higher place (with the Y value) and shift information technology a chip along the X-centrality every bit well. The Y-axis has the highest value. This ways that the meridian of the box receives the well-nigh light and it will be the shiniest side of the box.
The calorie-free is besides moved a scrap along the X-axis, so the right side of the box will also receive some light, but less.
And considering nosotros don't move the light position forth the Z-centrality, the front side of the box will not receive any light from this source. If there wasn't an ambient light, the front side would remain in darkness.
In that location are other light types likewise. The PointLight, for example, can exist used to simulate light bulbs. It has a fixed position and it emits light in every management. And the SpotLight tin can be used to simulate the spotlight of a car. It emits light from a single betoken into a direction forth a cone.
How to Prepare the Camera
So far, nosotros take created a mesh with geometry and material. And nosotros have also set upwards lights and added to the scene. We nonetheless need a camera to define how we wait at this scene.
There are two options here: perspective cameras and orthographic cameras.
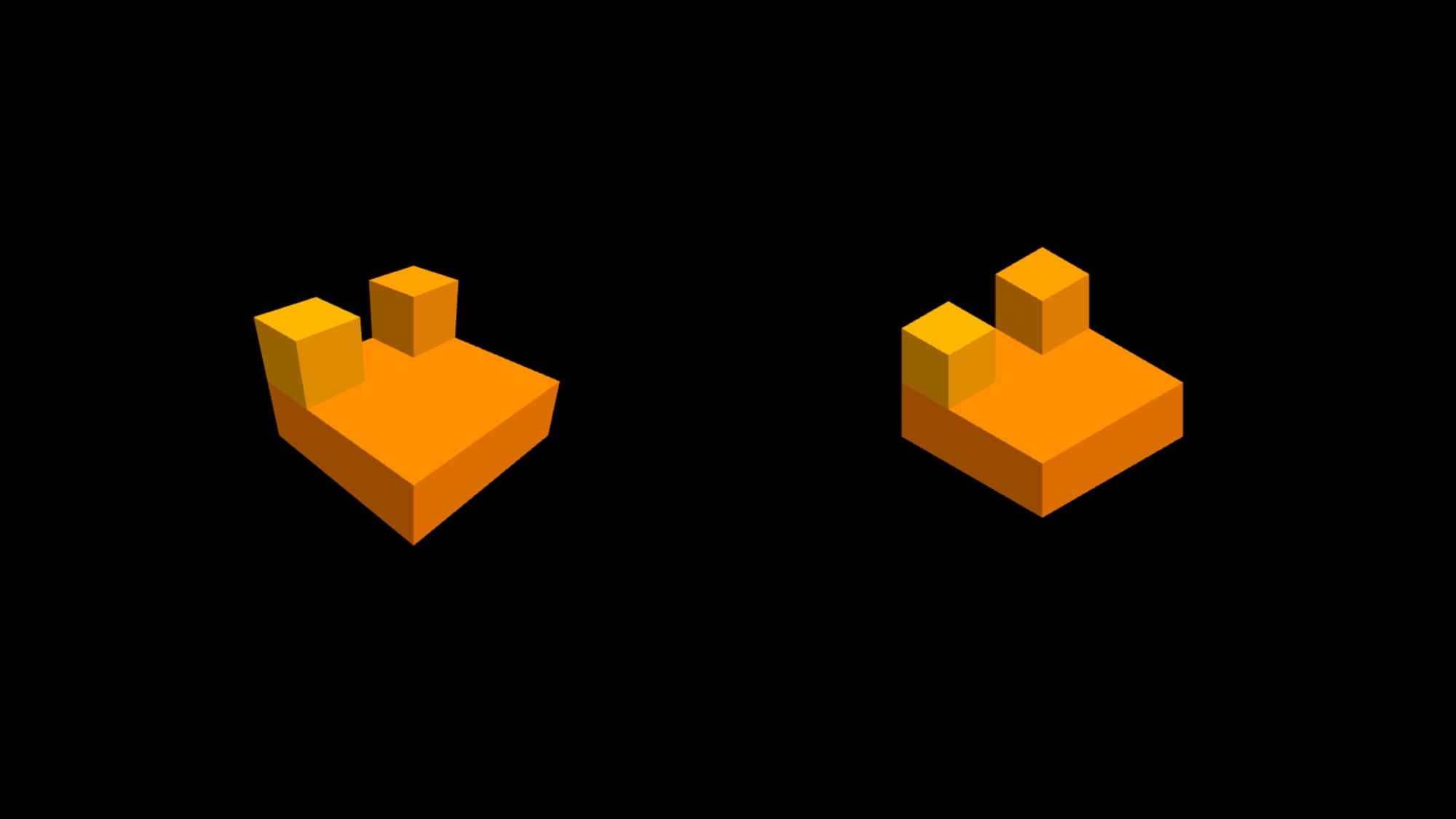
Video games mostly use perspective cameras, because how they work is similar to how you meet things in real life. Things that are further abroad appear to be smaller and things that are right in front of y'all appear bigger.
With orthographic projections, things volition accept the same size no matter how far they are from the camera. Orthographic cameras take a more minimal, geometric await. They don't distort the geometries - the parallel lines will appear in parallel.
For both cameras, nosotros have to define a view frustum. This is the region in the 3D infinite that is going to be projected to the screen. Anything exterior of this region won't appear on the screen. This is because it is either too close or too far away, or because the camera isn't pointed towards it.

With perspective project, everything within the view frustum is projected towards the viewpoint with a straight line. Things farther abroad from the camera appear smaller on the screen, considering from the viewpoint you lot can see them nether a smaller angle.
... // Perspective camera const attribute = window.innerWidth / window.innerHeight; const photographic camera = new THREE.PerspectiveCamera( 45, // field of view in degrees aspect, // aspect ratio 1, // near plane 100 // far aeroplane ); ...
To ascertain a perspective camera, you need to set a field of view, which is the vertical angle from the viewpoint. Then you define an aspect ratio of the width and the tiptop of the frame. If you fill the whole browser window and yous want to continue its attribute ratio, then this is how you can do information technology.
And then the last two parameters ascertain how far the near and far planes are from the viewpoint. Things that are too shut to the camera will exist ignored, and things that are too far away will be ignored as well.
... // Orthographic camera const width = 10; const top = width * (window.innerHeight / window.innerWidth); const camera = new Iii.OrthographicCamera( width / -two, // left width / two, // correct height / 2, // acme height / -2, // bottom 1, // near 100 // far ); ...
Then at that place'southward the orthographic camera. Here we are not projecting things towards a unmarried point but towards a surface. Each projection line is in parallel. That's why it doesn't matter how far objects are from the camera, and that's why it doesn't distort geometries.
For orthographic cameras, we take to define how far each aeroplane is from the viewpoint. The left plane is therefor five units to the left, and the right plane is v units to the correct, and and then on.
... camera.position.set(4, 4, 4); camera.lookAt(0, 0, 0); ...
Regardless of which camera are we using, we also need to position it and set it in a direction. If we are using an orthographic camera the actual numbers here don't matter that much. The objects will announced the same size no matter how far away they are from the camera. What matters, though, is their proportion.
Through this whole tutorial, we saw all the examples through the aforementioned camera. This camera was moved past the same unit forth every centrality and information technology looks towards the 0,0,0 coordinate. Positioning an orthographic camera is like positioning a directional calorie-free. It'south not the actual position that matters, but its direction.
How to Render the Scene
So nosotros managed to put together the scene and a photographic camera. Now only the final slice is missing that renders the paradigm into our browser.
We demand to ascertain a WebGLRenderer. This is the piece that is capable of rendering the bodily image into an HTML canvas when nosotros provide a scene and a camera. This is also where we can set the bodily size of this canvas – the width and height of the canvas in pixels as information technology should announced in the browser.
import * equally THREE from "three"; // Scene const scene = new Three.Scene(); // Add a cube to the scene const geometry = new THREE.BoxGeometry(iii, i, iii); // width, meridian, depth const material = new Iii.MeshLambertMaterial({ color: 0xfb8e00 }); const mesh = new Three.Mesh(geometry, material); mesh.position.set(0, 0, 0); scene.add(mesh); // Set lights const ambientLight = new 3.AmbientLight(0xffffff, 0.6); scene.add together(ambientLight); const directionalLight = new 3.DirectionalLight(0xffffff, 0.6); directionalLight.position.set up(10, 20, 0); // ten, y, z scene.add(directionalLight); // Camera const width = 10; const tiptop = width * (window.innerHeight / window.innerWidth); const photographic camera = new Three.OrthographicCamera( width / -two, // left width / 2, // right height / 2, // top height / -ii, // bottom 1, // near 100 // far ); camera.position.set(4, 4, 4); photographic camera.lookAt(0, 0, 0); // Renderer const renderer = new 3.WebGLRenderer({ antialias: true }); renderer.setSize(window.innerWidth, window.innerHeight); renderer.return(scene, camera); // Add it to HTML document.body.appendChild(renderer.domElement);
And finally, the concluding line here adds this rendered canvas to our HTML document. And that's all y'all need to render a box. It might seem a niggling as well much for just a single box, simply most of these things we only have to ready one time.
If y'all want to move forward with this projection, then check out my YouTube video on how to turn this into a simple game. In the video, we create a stack edifice game. We add game logic, effect handlers and blitheness, and even some physics with Cannon.js.
If you accept any feedback or questions on this tutorial, feel gratis to Tweet me @HunorBorbely or go out a comment on YouTube.
Larn to code for free. freeCodeCamp's open up source curriculum has helped more than than 40,000 people get jobs as developers. Get started
mansfieldhiceivien77.blogspot.com
Source: https://www.freecodecamp.org/news/render-3d-objects-in-browser-drawing-a-box-with-threejs/
0 Response to "why do i draw 3d boxes"
Enviar um comentário